C++ Variant Index
It is type-safe and knows what type it is, and it carefully constructs and destroys the objects within it when it should.
C++ variant index. The following example shows how to clear an array of variants, where celt is the number of elements in the array. To enable such retargeting from a single implementation, the system relies on C++ templates and metaprogramming. Access std::variant by index.
To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store:. If our container needs only contain basic types and Plain Old Data Structures, the solution is simple:. 2 minutes to read;.
Unreal Engine 4 Documentation > Unreal Editor Manual > Levels > Working with Scene Variants > Calling Functions on Variant Activation. I want to access mail items when sending mail so that i need to get body, To, Cc, subject & attachments of mail from outlook before sending mail using C++?. There is a low chance that we will regret including variant in C++17, but a high chance that we will regret omitting it.
The type must be unique and the index valid. If the visitor approach is acceptable but has_alternative is not, get<> also accepts index values (as numeric template parameters, e.g., get<0>(v), get<1>(v), etc.) and variant has a method called index() to give a numeric value saying what the current type is. ClassArray.erase(classArra y.begin()+ index);//r emove an object from the array at index //if an in vector has these elemntes 5 9 8 6 4 10 2, then erasing an int at index 2 will make the vector like this 5 9 6 4 10 2.
Notable features of boost::variant include:. This overload only participates in overload resolution if std::is_constructible_v<T. Attempts to do so will return an HRESULT containing DISP_E_ARRAYISLOCKED.
In the post C++17 - What's New in the Library are the details to the three data types that are part of C++17. It also knows the type of the value it currently contains (if you’ve ever implemented a type-safe union, you’ll realize why that part is important). Consider the array x we have seen above.
C++17 In Detail by Bartek!. In the interface of std::variant, the index is the number that defines which of the alternative types are stored in the variant. After the assignment with the double, the index is 1.
There’s a little trick hiding in the default case:. Contains key (True or False) Input :. Elements of an array in C++ Few Things to Remember:.
But you have to keep a few rules in mind. C++ allows namespace level constants, variables, and functions. Unlike VS 15’s Updates (which combined IDE and toolset changes),.
The C++ community should not wait three years for a widely useful library that is already done, fits its purpose, and has had such extensive review. A function template behaves like a function except that the template can have arguments of many different types (see example). Since the class std::vector is basically a class that manages a dynamically allocated contiguous array, the same principle explained here applies to C++ vectors.
C++ Array is the collection of items stored at contiguous memory locations. Variant is a replacement for raw union use. Specifically, converting constructors, assignment from values, assignment from variants, swapping, and construction from string literals are all failing.
Returns the zero-based index of the alternative that is currently held by the variant. Returns the zero-based index of the alternative that is currently held by the variant. In this implementation, we want to switch on v.index() instead and invoke f(std::get<I>(v)) directly rather than through a function.
That means that, for example, five values of type int can be declared as an array without having to declare 5 different variables (each with its own identifier). Visual Studio 17 version 15.3 preview is now available, containing an updated Visual C++ toolset (i.e. Where I is the zero-based index of T in Types.
First occurrence of key (index of array). Replacing Unique_ptr With C++17's std::variant — a Practical Experiment - DZone IoT IoT Zone. Phew, what a mouthful!.
This proposal attempts to apply the lessons learned from optional (1). Essentially, the VARIANT structure is a container for a large union that carries many types of data. A Note on Efficiency.
In earlier versions of C++ they are always non-variadic. Since C++11, we can actually make this code work by either proving a defaulted default constructor for the non-POD type or provide a proper constructor ( and destructor ) to the union which will handle properly the. Therefore, I get a std::bad_variant_access exception.
Boost::variant is defined in boost/variant.hpp.Because boost::variant is a template, at least one parameter must be specified. Std::visit is a way to invoke an operation on the currently active type in the variant. Std::variant< int, std::string > var;.
How to access these functions using C++?. In this example, x5 is the last element. For simplicity reason, I will refer only to std.
The following program, compiled in Builder 10.3 as a console-mode program with the default compiler, illustrates the situation. As with unions, if a variant holds a value of some object type T, the object representation of T is allocated directly within the object representation. We can store one of either type in it:.
The value in the first member of the structure, vt, describes which of the union members. When programming in C++ we sometimes need heterogeneous containers that can hold more than one type of value (though not more than one at the same time). Meaning x0 is the first element stored at index 0.
Std::visit not only can take many variants, but also those variants might be of a. Std::variant is a type-safe union. In_place in_place_type in_place_index in_place_t in_place_type_t in_place_index_t.
Monostate > can be used instead). All of the instructions that you'll need to use these functions can be found in the comments below. // syntax to access array elements arrayindex;.
(GetVariant) values from a variant safe array. Std::variant appears to be massively broken. Variant is a data type in certain programming languages, particularly Visual Basic, OCaml, Delphi and C++ when using the Component Object Model.
An array is a series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding an index to a unique identifier. Most of the Microsoft Active Accessibility functions and the IAccessible properties and methods take a VARIANT structure as a parameter. In Example 24.1, v can store values of type double, char, or std::string.However, if you tried to assign a value of type int to v, the resulting code would not compile.
Function templates, class templates and, since C++14, variable templates.Since C++11, templates may be either variadic or non-variadic;. If the variant is valueless_by_exception , returns variant_npos. That case gets hit either when the index is 2, indicating that we have a std:string, or when the index is variant_npos, indicating that the variant is in a horrible state.
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. STIX (Structural Variant Index) supports searching every discordant paired-end and split-read alignment from thousands of sample BAMs or CRAMs for the existence of an arbitrary SV. All such Java declarations must be inside a class or interface.
(since C++17) Returns the zero-based index of the alternative that is currently held by the variant. A variant is permitted to hold the same type more than once, and to hold differently cv-qualified versions of the. So, in the little example above, the index of io is 0 after the construction, because std::vector<int> is the first type in the list.
So accessing a VARIANT array in C++ involves interpreting the contents of the VARIANT as a pointer to a SAFEARRAY, validating the first array index against cDims to be sure it's in range, then indexing into pvData by the size of cbElements, accounting for the contents of this indices entry in the rgsabound array. Visit( (auto&& e) { std::cout << e << '\n';. Differences between C++ and Java are:.
Tuple (C++11) apply (C++17) make_from_tuple. Whereas standard containers such as std::vector may be thought of as "multi-value, single type," variant is "multi-type, single value.". When you activate a Variant, call a function instead of changing a property value.
For single visitation in Variant Visitation, we generate a 1-dimensional array of function pointers where the function pointer at I performs f(std::get<I>(v)). Like VS 15’s Updates, we’re adding C++17 features in VS 17’s Updates, at a similar release frequency. We can then invoke the function pointer at v.index().
If the variant is valueless_by_exception , returns variant_npos. A provided type, monostate, can be used as the first type in those scenarios.variant<monostate, std::mutex, std::recursive_mutex> will default-construct a monostate, which is basically a no-op, as monostate is. C++17 STL Cookbook by Jacek Galowicz;.
However, in cases such as variant<std::mutex, std::recursive_mutex>, one might legitimately wish to avoid constructing a std::mutex by default. The variant class template is a safe, generic, stack-based discriminated union container, offering a simple solution for manipulating an object from a heterogeneous set of types in a uniform manner. This is usually the desired behavior.
The module centres around the Variant type:. C++ Fundamentals Including C++ 17 by Kate Gregory;. One or more template parameters specify the supported types.
Simply copy and paste the code from this article into your files and follow these instructions. STIX reports a per-sample count of all concurring evidence. The class template std::variant represents a type-safe union.An instance of std::variant at any given time either holds a value of one of its alternative types, or it holds no value (this state is hard to achieve, see valueless_by_exception).
C++17 - The Complete Guide by Nicolai Josuttis;. What does construction in place mean?. Implementing a variant type from scratch in C++.
This is not actually a sum type like C++’s std::variant, but a type-safe container that can contain a value of any type. A variant is not permitted to hold references, arrays, or the type void. C++ parsing is somewhat more complicated than with Java;.
Is a sequence of comparisons if Foo is a variable, but it creates an object if Foo is the name of a class template. On line 19, the variant w holds an int value. Edit Example.
You can ask for the value of a variant by type (line 15) or by index (line 16). Empty variants are also ill-formed (std::. The array is the series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding the index to a unique identifier.
It’s a callable object with overloads for all the possible types in the variant(s). While the use of not explicitly declared variants is not recommended,. And we can access the contents via std::visit:.
Std::any is a type that may hold an object of an arbitrary type. To check the currently active type you can use std::holds_alternative or std::variant::index;. Variant is not allowed to allocate additional (dynamic) memory.
Visual C++ / C++. In Visual Basic the Variant data type is a tagged union that can be used to represent any other data type except fixed-length string type and record types. This is similar to.
The array indices start with 0. Accessing the vector's content by index is much more efficient when following the row-major order principle. Thanks in advance Swathi Here is my Code:.
Resources about C++17 STL:. Compiler, linker, and libraries). If the size of an array is n, the last element is stored at index (n-1).
Variants containing arrays with outstanding references cannot be cleared. There are three kinds of templates:. Practical C++14 and C++17 Features - by Giovanni Dicanio;.
Variants in C++ ¶ As described in the section on choosing variants, Mitsuba 2 code can be compiled into different variants, which are parameterized by their computational backend and representation of color. Call to implicitly-deleted default constructor of ‘union (anonymous union at variant.cpp:4:1)’ } foo;. This creates a variant (a tagged union) that can store either an int or a string.
So what you want is something that can either hold a single value or a std::vector of values, with the idea that you don't want to pay for the memory and/or CPU overhead of a std::vector for a uniform field. In order not to repeat me. _IDTExtensibility2 methods can able to access but _MailItem methods not able to access.
In Visual Basic, any variable not declared explicitly or the type of which is not declared explicitly, is taken to be a variant. In line 11-13 you see three possibilities to assign the variant v the variant w. 2 3 3 5 5 5 6 6 Function :.

Index Apache Activemq Cpp Apache Software Foundation

New Wine In Old Bottle New Azorult Variant Found In Findmyname Campaign Using Fallout Exploit Kit
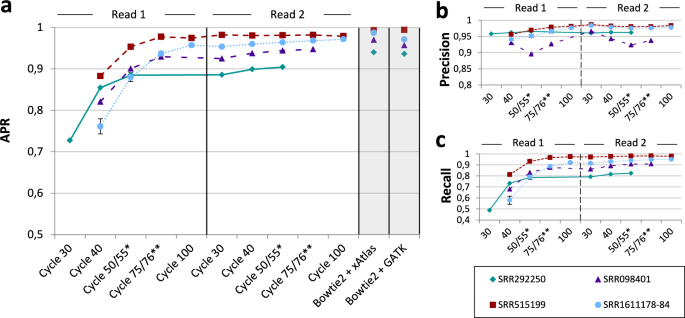
Phra0bj6qzamjm
C++ Variant Index のギャラリー
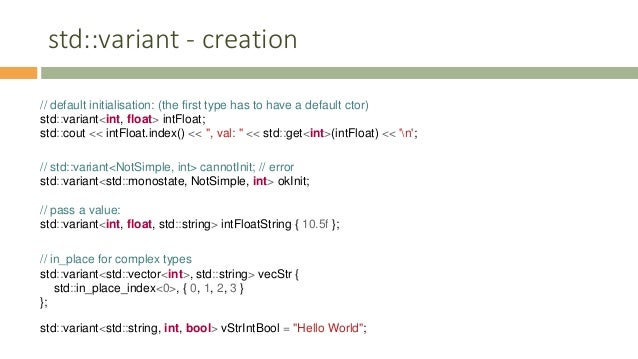
Vocabulary Types In C 17
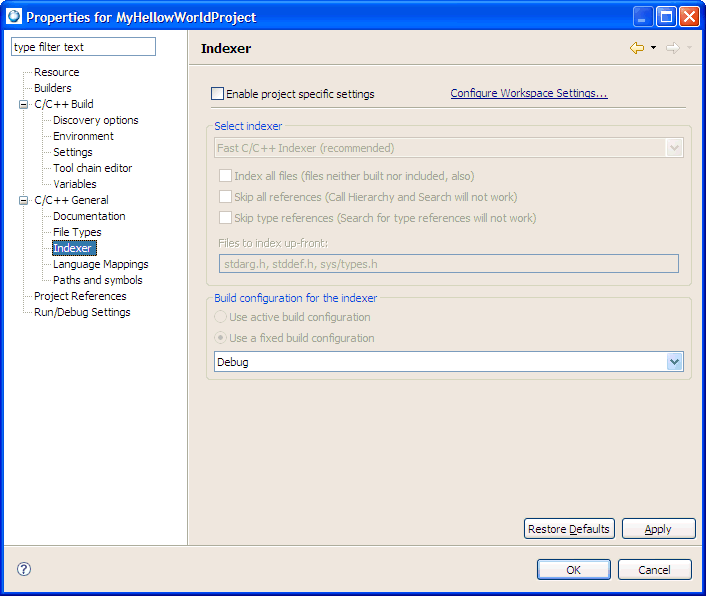
Creating A C C Project

Demonstrating The Utility Of Flexible Sequence Queries Against Indexed Short Reads With Flextyper Biorxiv
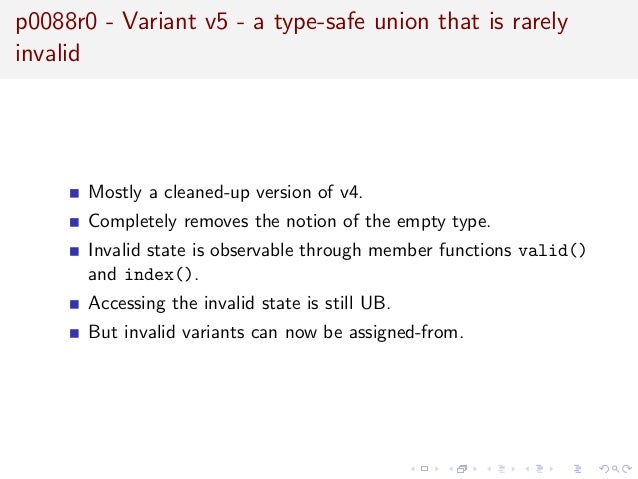
Discriminating Unions The Long Road To Std Variant
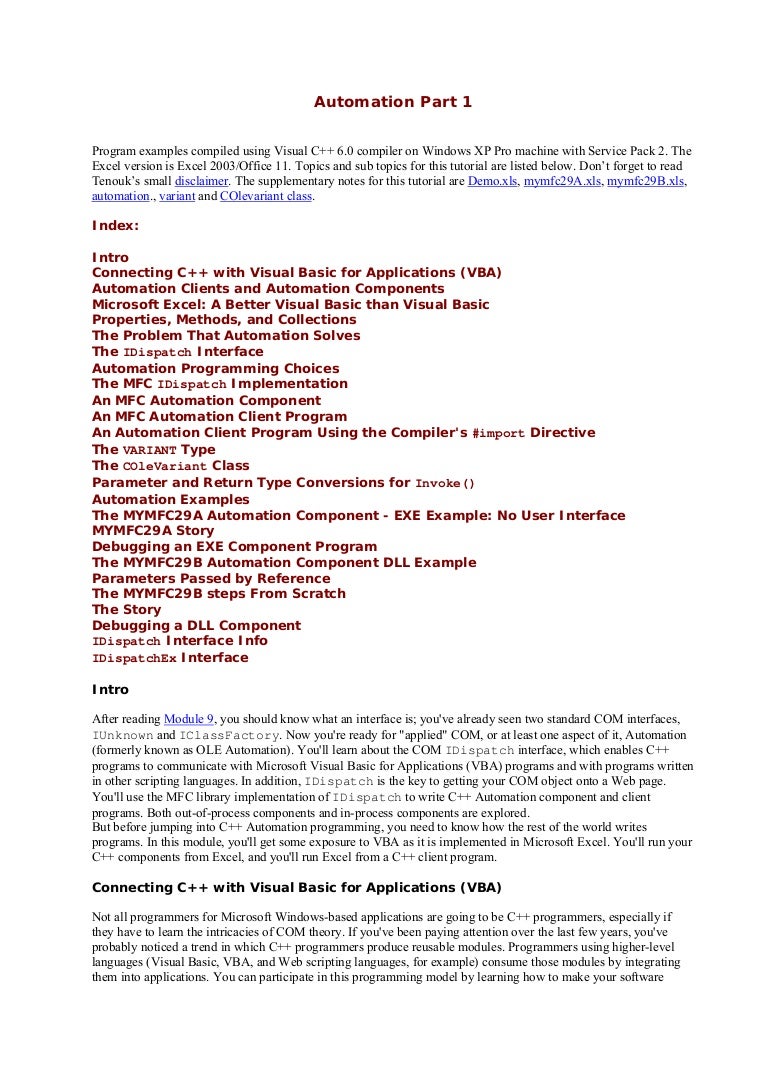
Mfc Programming Tutorial Automation Step By Step
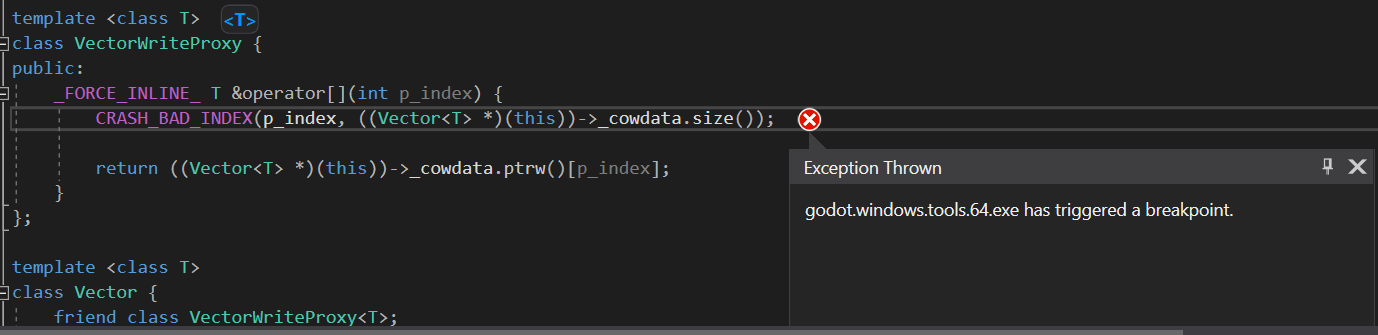
Saving Scripts That Use Tool Keyword Can Crash The Editor Issue Godotengine Godot Github

C 17 Std Variant Rangarajan Krishnamoorthy On Programming And Other Topics

Eclipse Cdt Does Not Parse Variant Include File Correctly Stack Overflow

Variant Condition Propagation With Variant Sources And Sinks Matlab Simulink

A New Azorult C Variant Can Establish Rdp Connections Informatica

C 17 Std Variant Rangarajan Krishnamoorthy On Programming And Other Topics
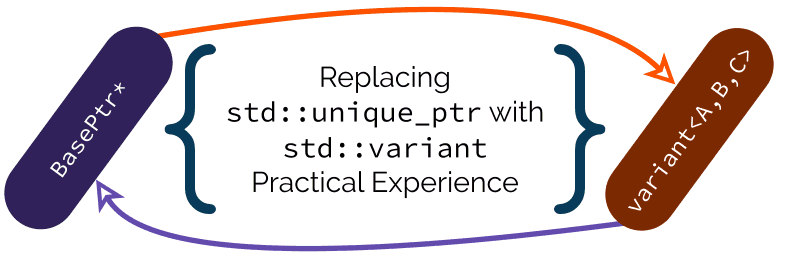
3am4ai6iy3abcm

A Wavelet Tree Based Fm Index For Biological Sequences In Seqan January 30 12 Myslide 专注ppt分享 追随slideshare和speakerdeck的脚步
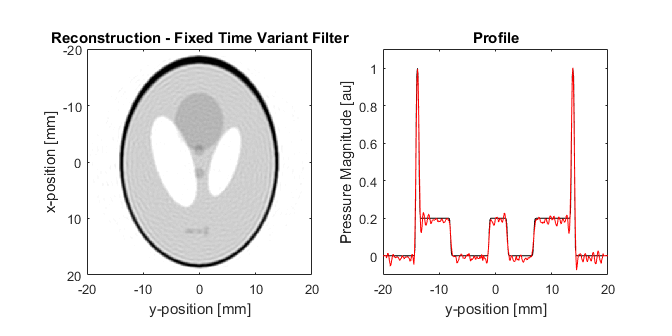
K Wave Matlab Toolbox
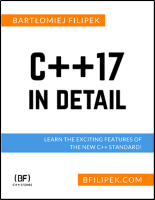
Bartek S Coding Blog Everything You Need To Know About Std Variant From C 17

Variant Kudu An Efficient Tool Kit Leveraging Distributed Bitmap Index For Analysis Of Massive Genetic Variation Datasets Journal Of Computational Biology

Stl Header Token Parsing Benchmarks For Vs08 Vs12 Vs13 And Vs17 Cpp
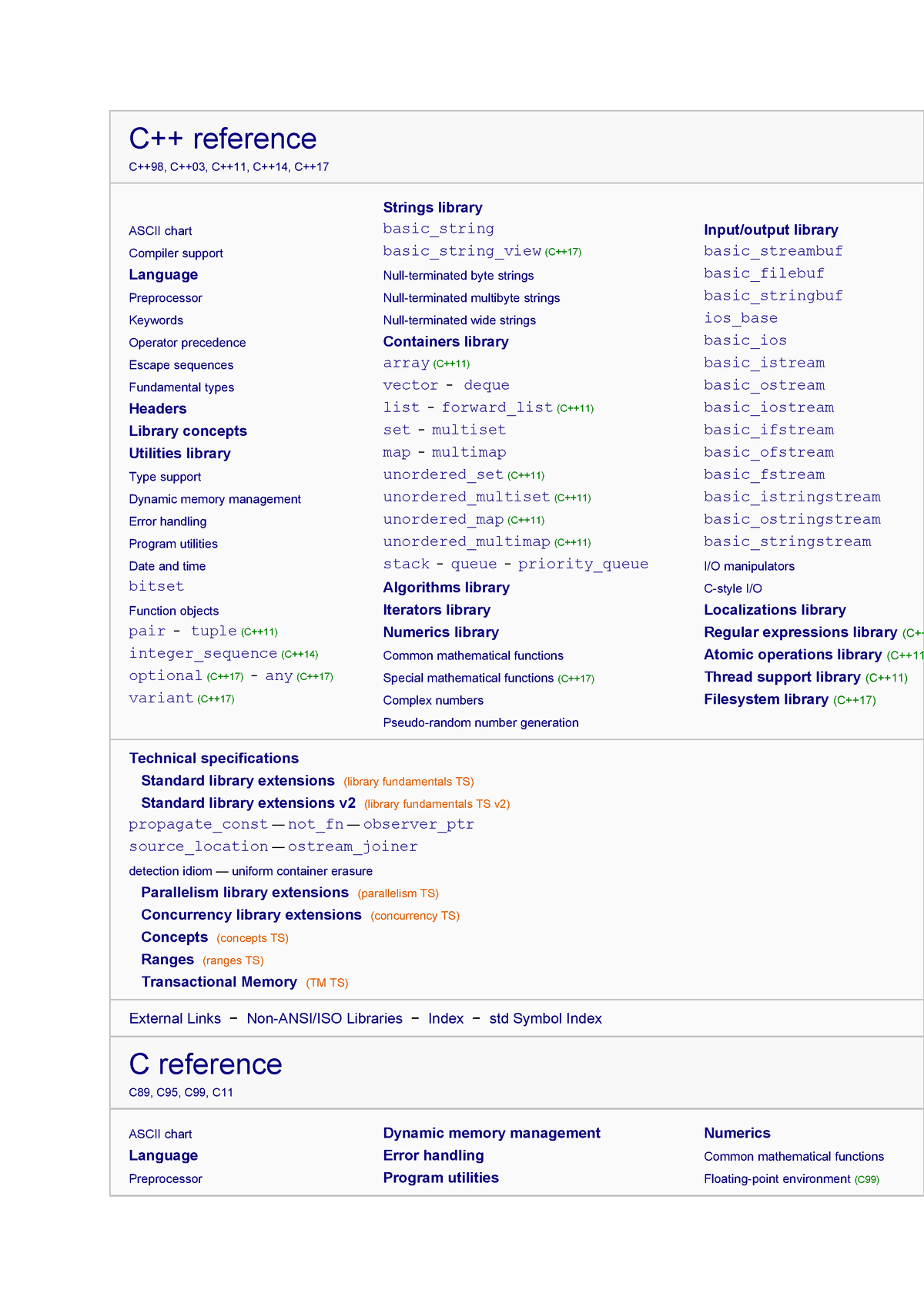
C Reference Notes Csc 131 Computational Thinking Msu Studocu
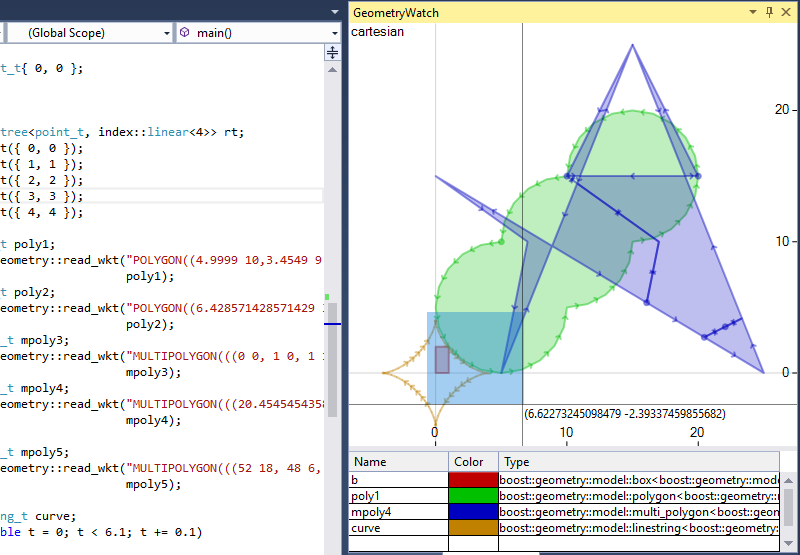
Graphical Debugging Visual Studio Marketplace

C Core Guidelines Type Safety By Design Modernescpp Com

October 18 Reverse Engineering Malware Deep Insight

Clingen Allele Registry Links Information About Genetic Variants Pawliczek 18 Human Mutation Wiley Online Library
Github Martinmoene Variant Lite Variant Lite A C 17 Like Variant A Type Safe Union For C 98 C 11 And Later In A Single File Header Only Library

Combining Universal And Existential Quantification Download Scientific Diagram

The Best Visual Studio Code Dark And Light Themes Updated August 19 By Shu Uesugi Medium

Std Variant Vs Inheritance Vs Other Ways Performance Stack Overflow

Using The String To Integer Index Download Scientific Diagram

Nprg051 Pokrocile Programovani V C Advanced C Programming Ppt Download

Variant Manager Overview Unreal Engine Documentation
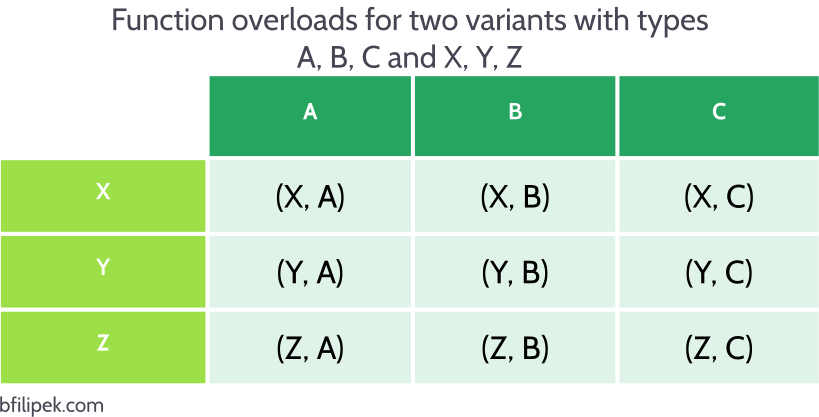
Bartek S Coding Blog How To Use Std Visit With Multiple Variants
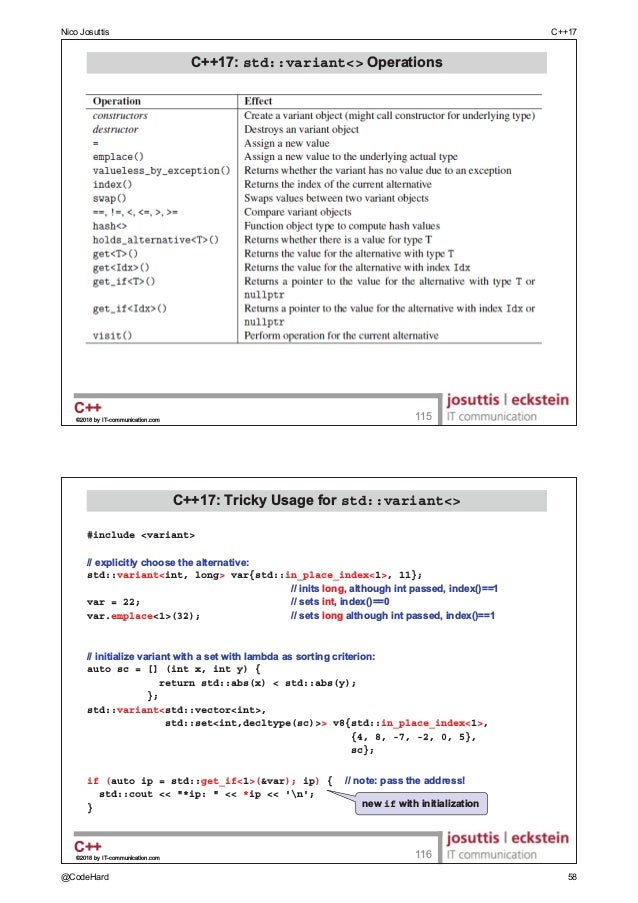
Beware Of C 17

Variant Manager Overview Unreal Engine Documentation

Eclipse Cdt Does Not Parse Variant Include File Correctly Stack Overflow
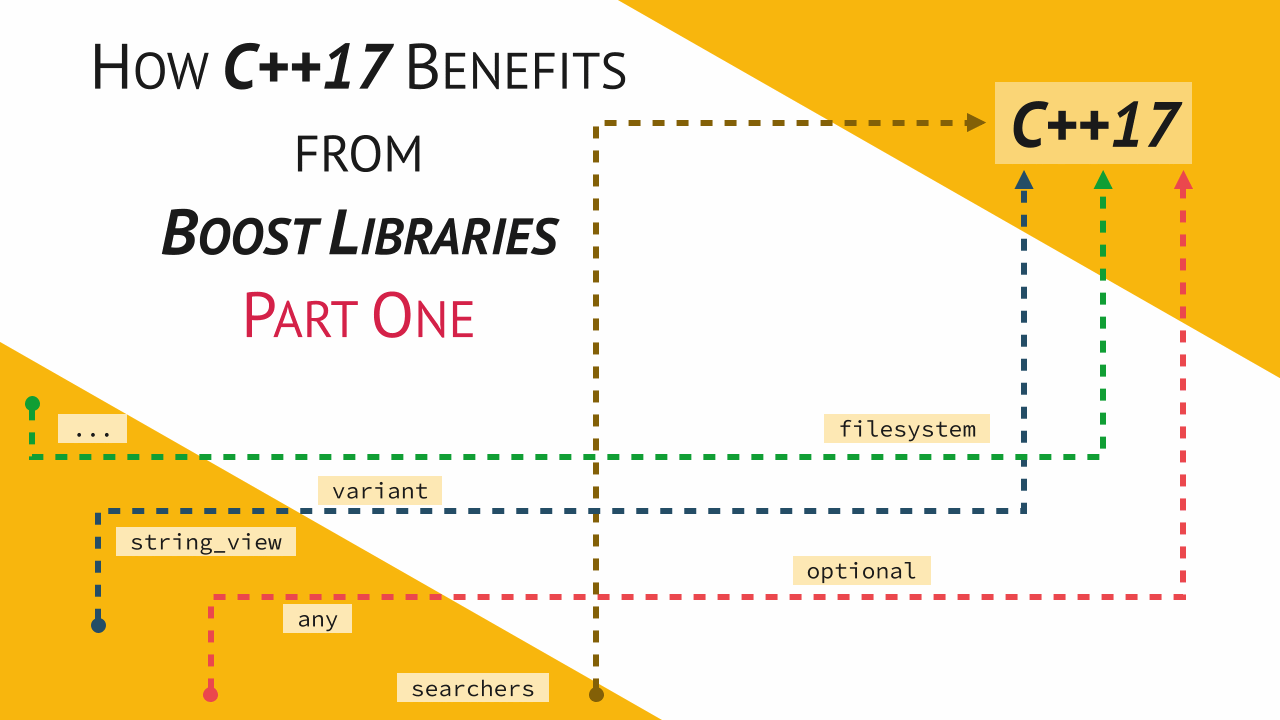
How C 17 Benefits From Boost Libraries Part One Fluent C
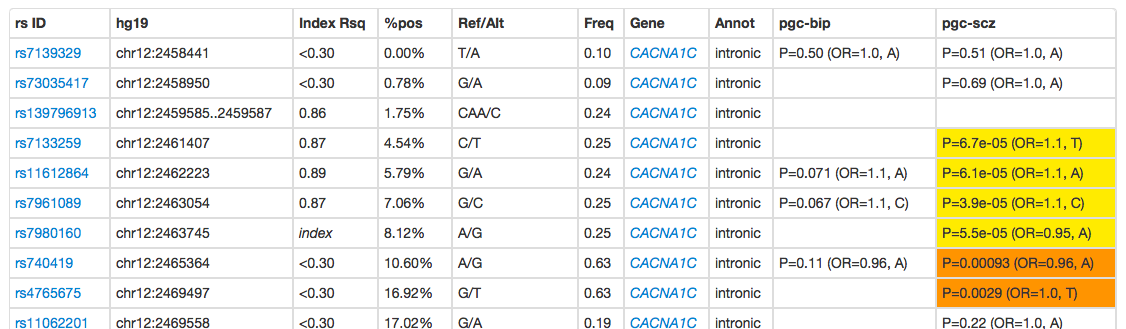
Ldookup Help
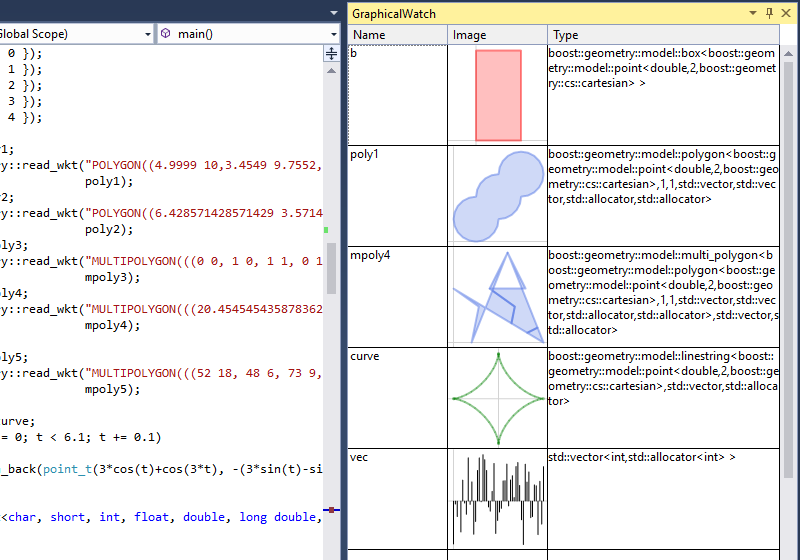
Graphical Debugging Visual Studio Marketplace

Cr5 Tnzul8ddqm
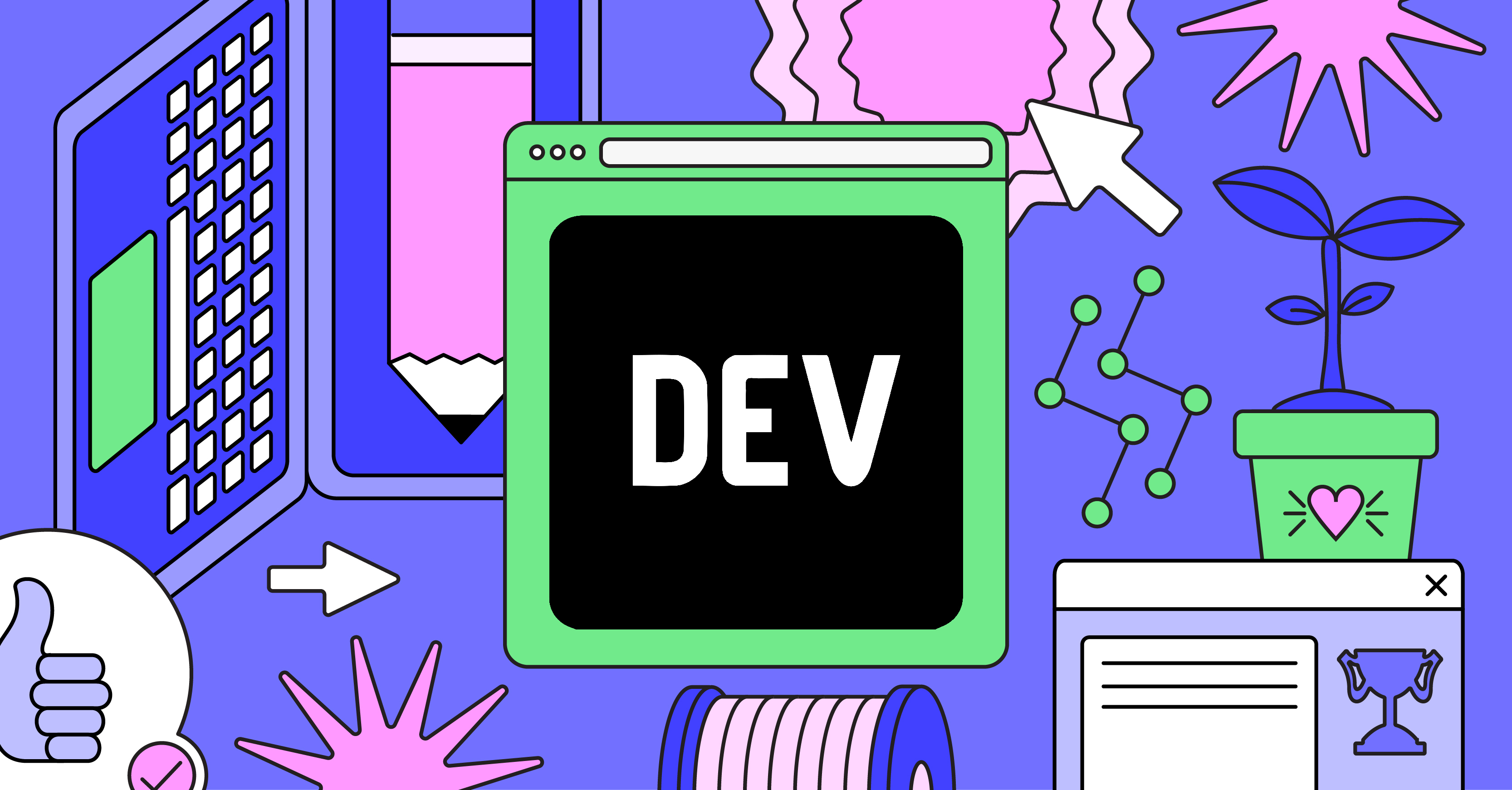
Let S Write ged Union In C Dev
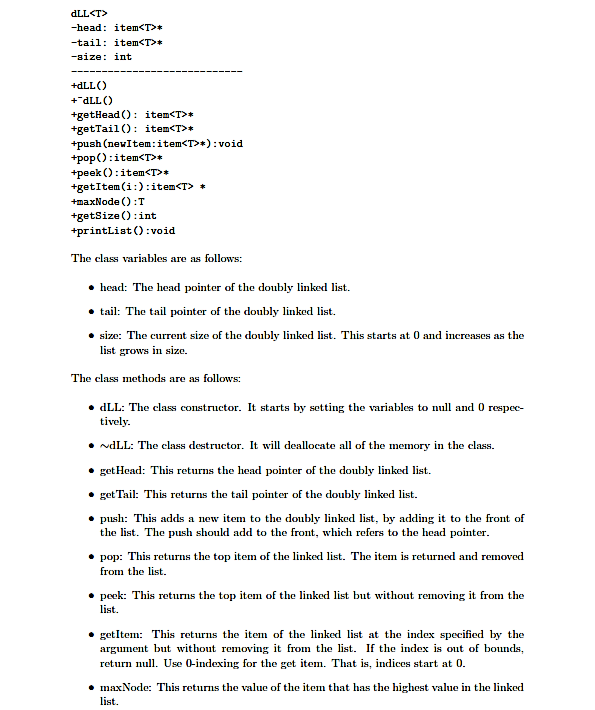
In C Use Doubly Linked List To Write The Followi Chegg Com

Std Variant Vs Inheritance Vs Other Ways Performance Stack Overflow
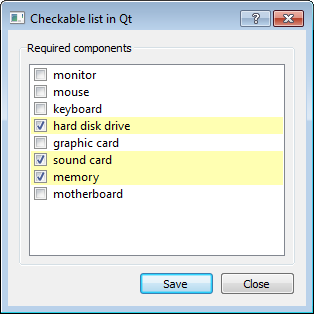
Checkable List In Qt With Qlistwidget Or Qlistview

Plot Sample Mathgl 2 4 4
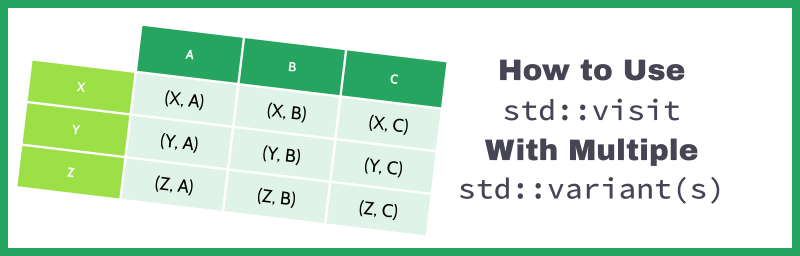
Bartek S Coding Blog How To Use Std Visit With Multiple Variants
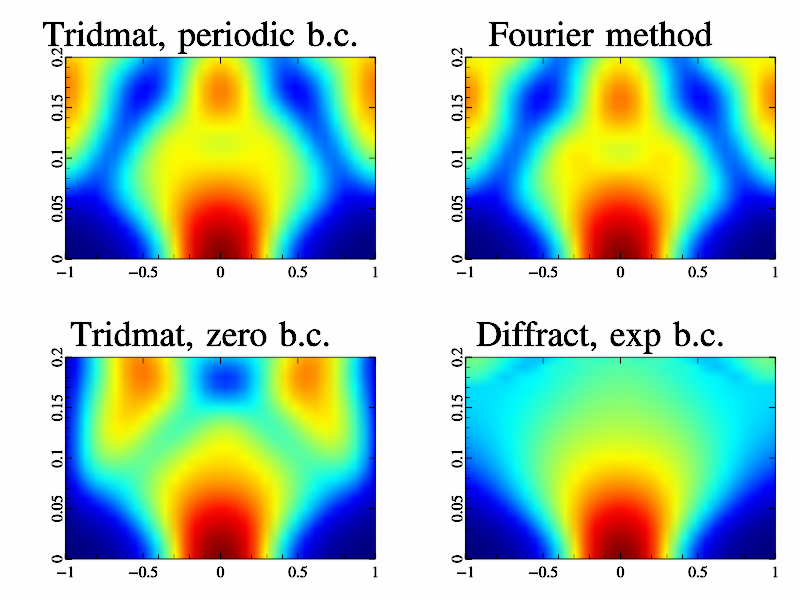
Diffract Sample Mathgl 2 4 4

Variant Manager Overview Unreal Engine Documentation

Ldookup Help
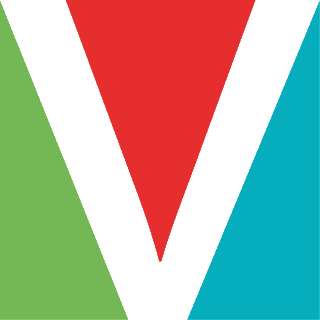
Vittorio Romeo S Website
Www Sciencedirect Com Science Article Pii S Pdf Md5 B0abb4ee048ddb12a5 Pid 1 S2 0 S Main Pdf Valck 1
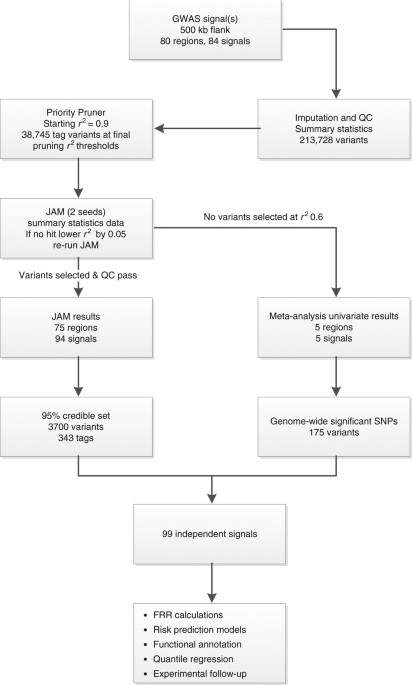
Fine Mapping Of Prostate Cancer Susceptibility Loci In A Large Meta Analysis Identifies Candidate Causal Variants Nature Communications
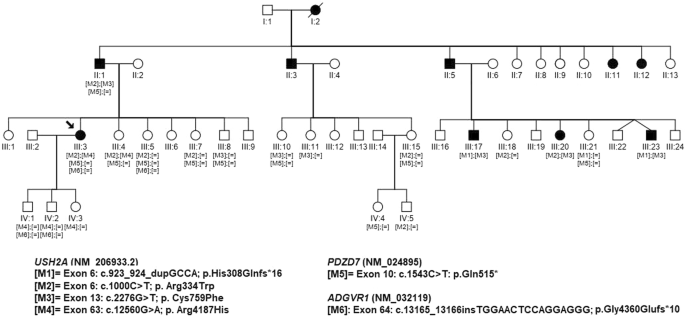
Unmasking Retinitis Pigmentosa Complex Cases By A Whole Genome Sequencing Algorithm Based On Open Access Tools Hidden Recessive Inheritance And Potential Oligogenic Variants Springerlink

Variant Manager Overview Unreal Engine Documentation

Clingen Allele Registry Links Information About Genetic Variants Abstract Europe Pmc

C Core Guidelines Type Safety By Design Modernescpp Com
C 17 What S New In The Library Modernescpp Com
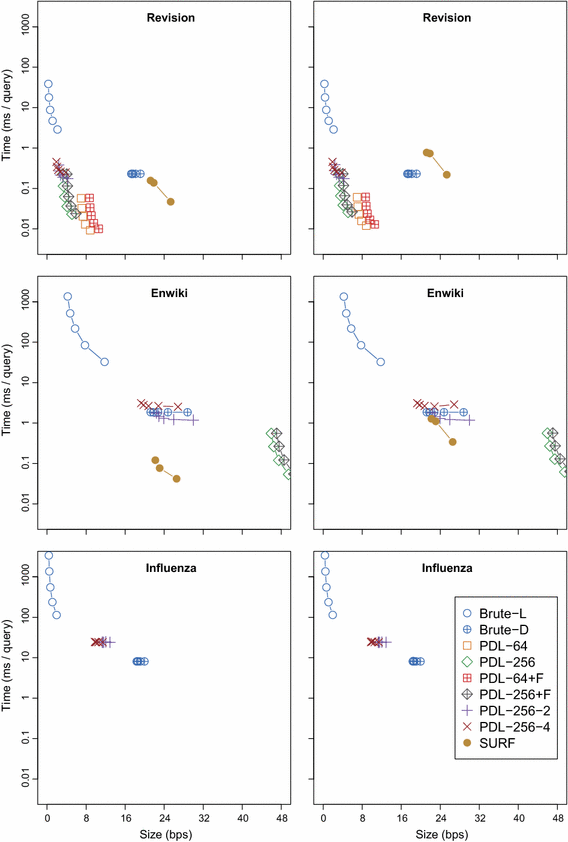
Document Retrieval On Repetitive String Collections Springerlink
Choosing Variants Mitsuba2 0 1 Dev0 Documentation

Creating Qml Properties Dynamically At Runtime From C Machine Koder
I Alway Cannot Get The Correct Data By Safearraygetelement
Www Sciencedirect Com Science Article Pii S Pdf Md5 B0abb4ee048ddb12a5 Pid 1 S2 0 S Main Pdf Valck 1
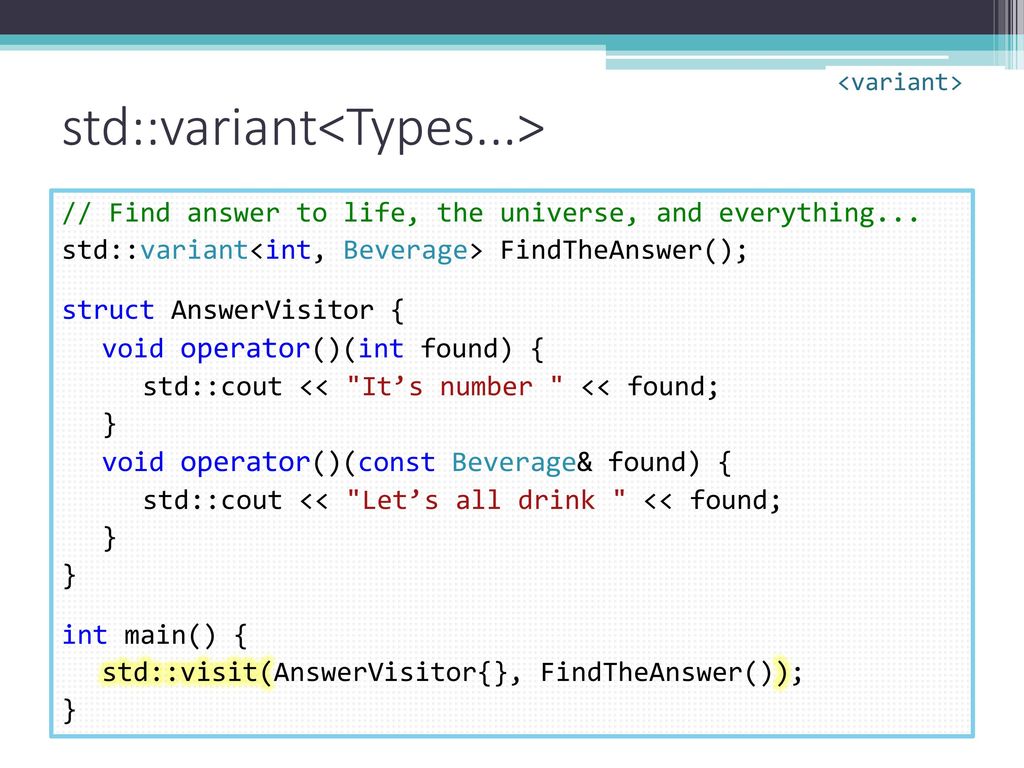
Part Ii The Standard Library Ppt Download

Variantmetacaller Automated Fusion Of Variant Calling Pipelines For Quantitative Precision Based Filtering Topic Of Research Paper In Biological Sciences Download Scholarly Article Pdf And Read For Free On Cyberleninka Open Science Hub

Pacific 17 Nick Sarten Type Safe State Machines With C 17 Std Variant Youtube
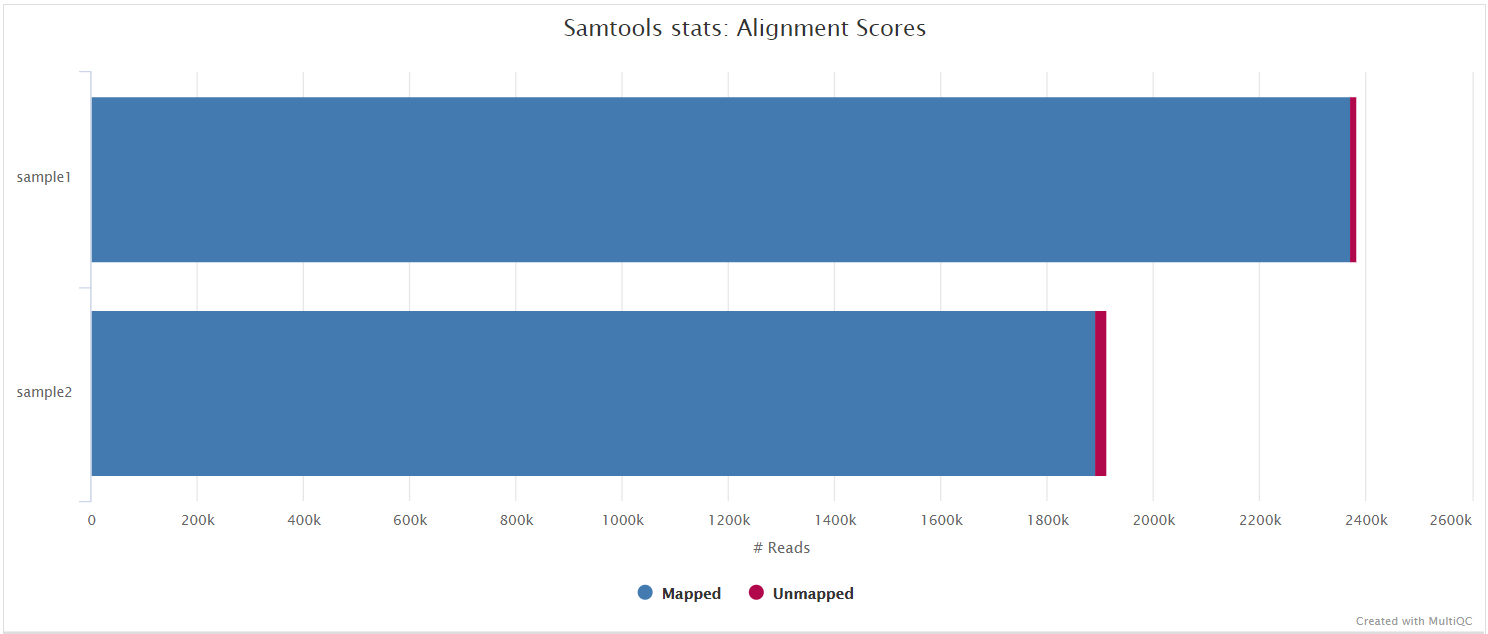
Viralrecon Nf Core

Frans Bouma C S Variant Does The Same The Int Argument Is A Start Index It Scans Backwards Btw The Toindex Variant You Mention Is An Overload With Fromindex And Toindex
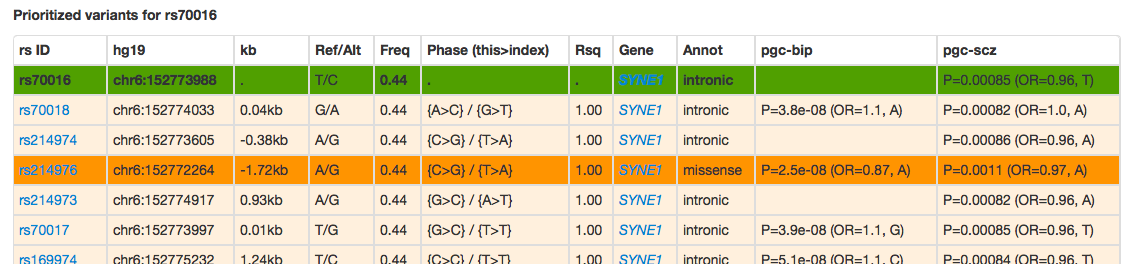
Ldookup Help

C 17 Std Variant Rangarajan Krishnamoorthy On Programming And Other Topics
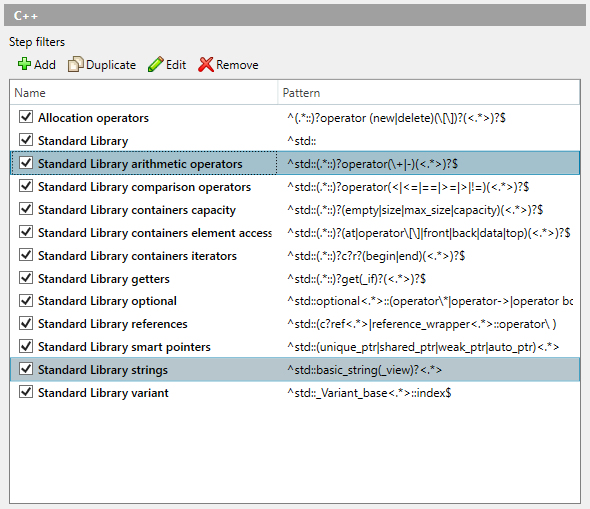
What S New In Resharper C 18 1 Resharper C Blog Jetbrains
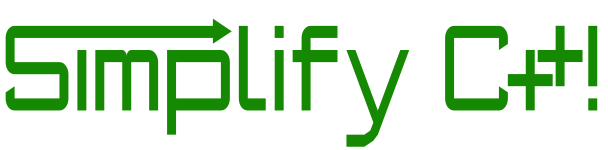
Modern C Features Std Variant And Std Visit Simplify C
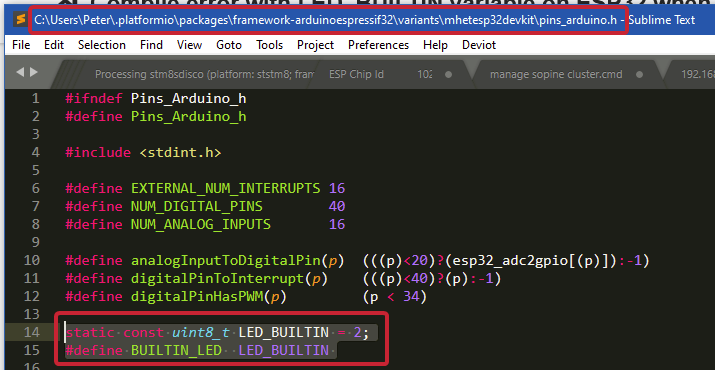
Compile Error With Led Builtin Variable On Esp32 When Moving Source From Arduinoide Development Platforms Platformio Community

Variant Condition Propagation With Variant Sources And Sinks Matlab Simulink
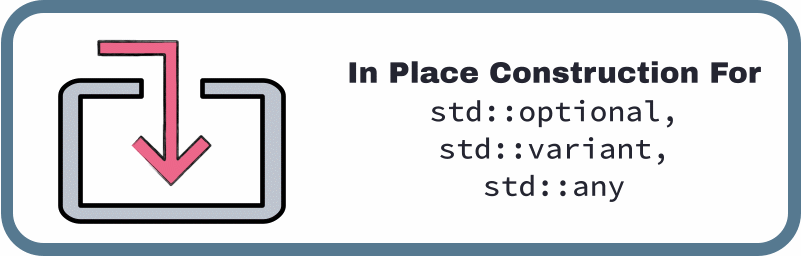
In Place Construction For Std Any Std Variant And Std Optional C Stories

Material Ui You Are Using The Typography Variant

C 17 What S New In The Library Modernescpp Com
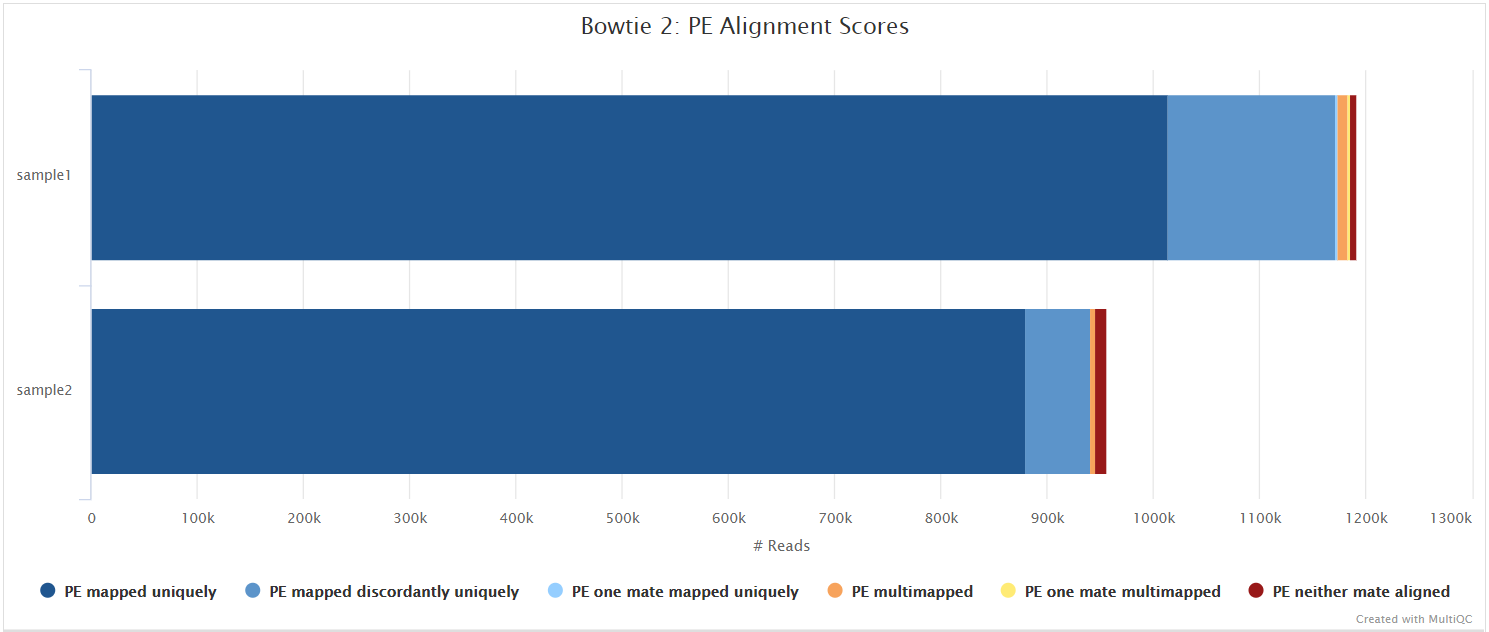
Viralrecon Nf Core
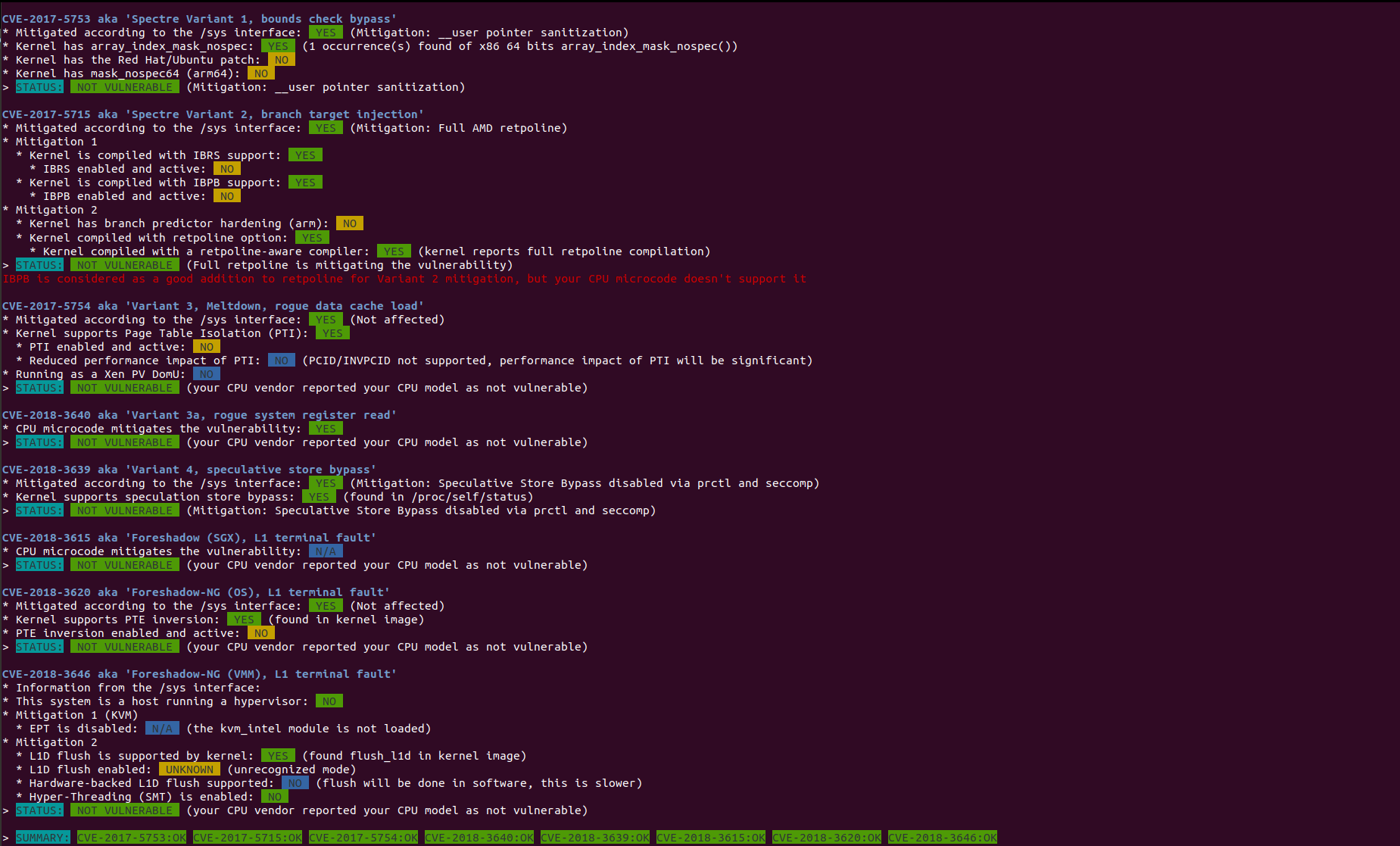
Thoughts Dereferenced From The Scratchpad Noise Meltdown And Spectre On Pc Engines Apu2
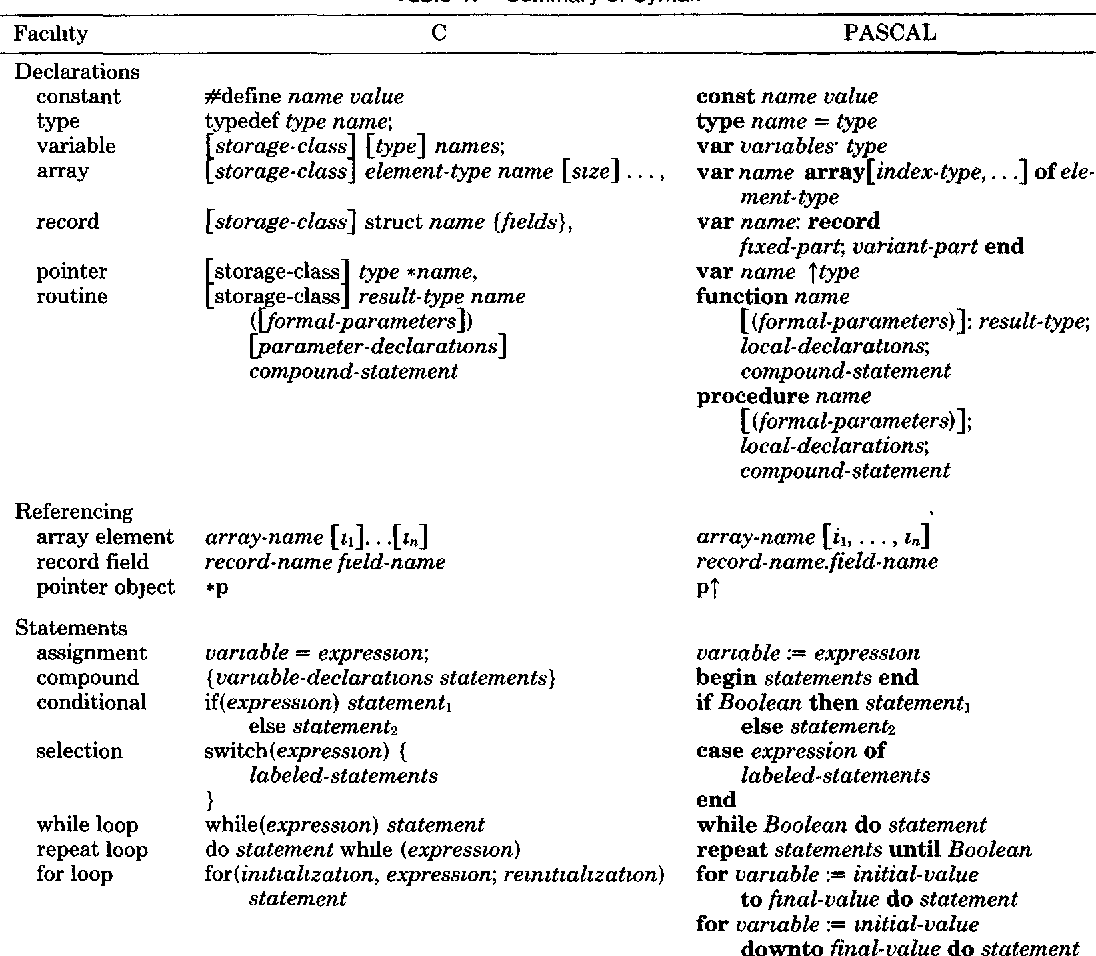
Comparison Of The Programming Languages C And Pascal Semantic Scholar
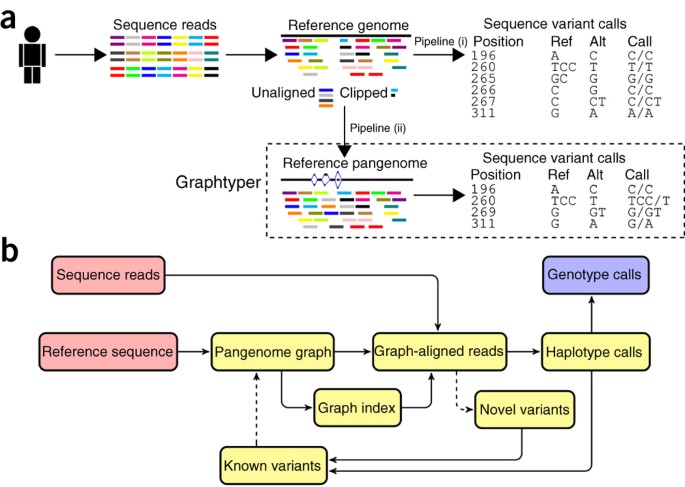
Graphtyper Enables Population Scale Genotyping Using Pangenome Graphs Nature Genetics
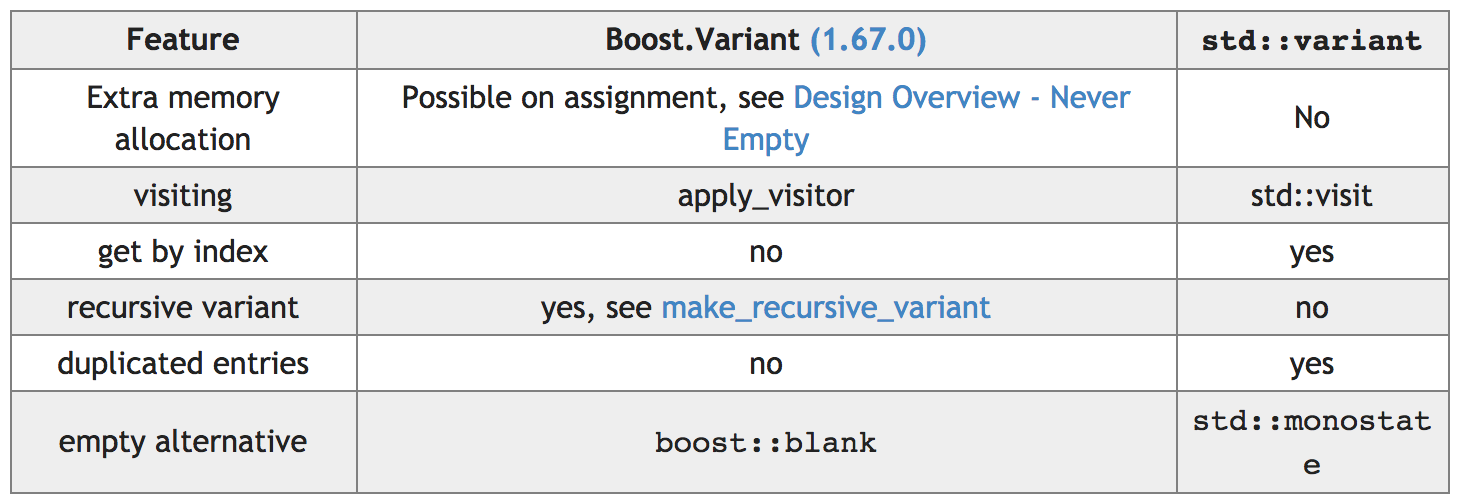
C Annotated June September 18 Clion Blog Jetbrains
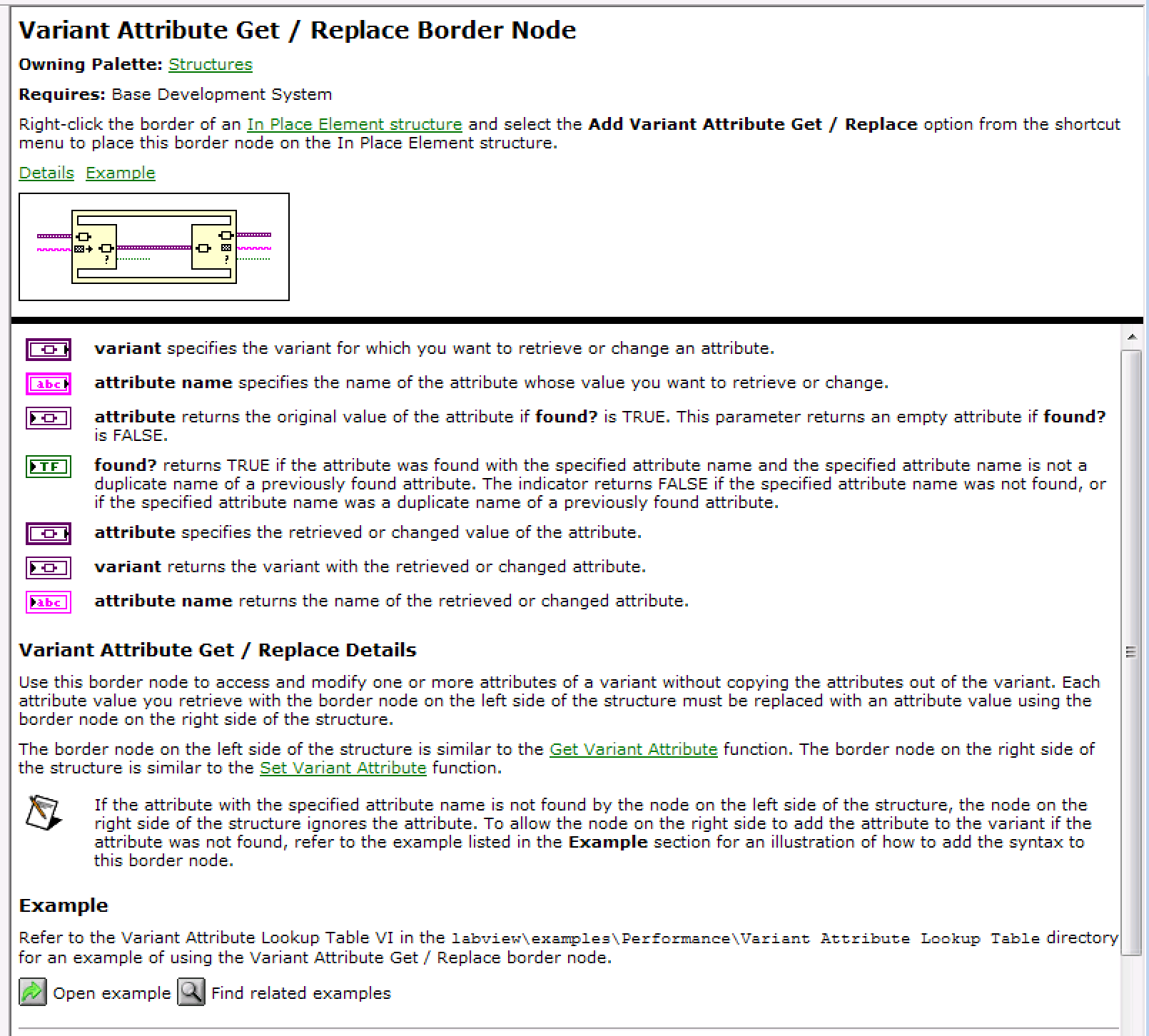
Using Variant Attributes For High Performance Lookup Tables In Labview Ni Community National Instruments
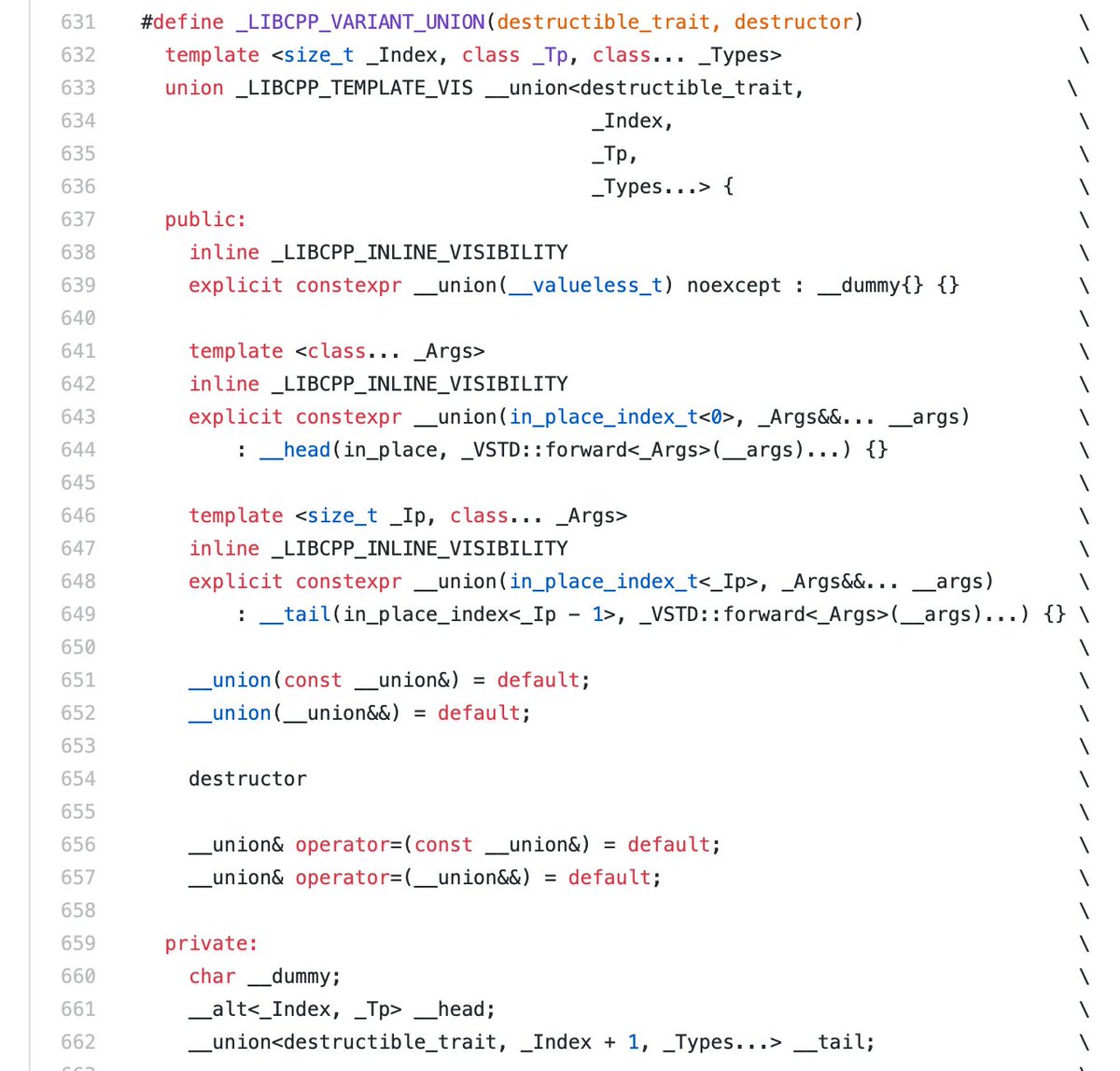
Shafik Yaghmour A Twitter Til Well Ok Recently At Least Variadic Unions Are A Thing Template Size T I Typename T Typename Types Union V I T Types T Head V I 1 Types Tail
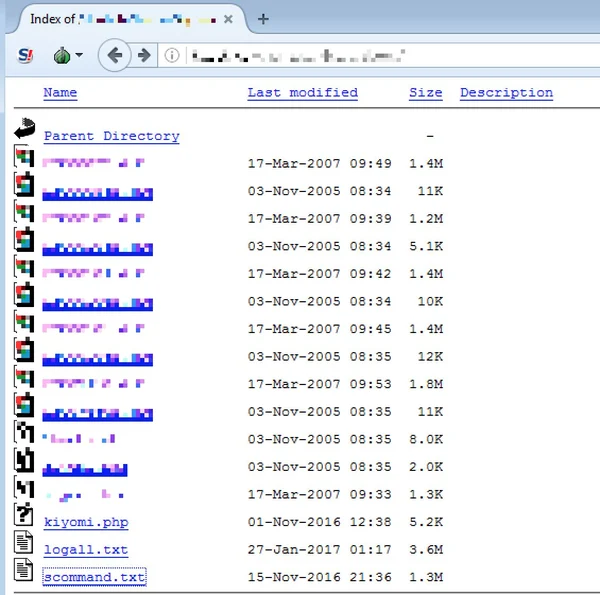
Shadowwali New Variant Of The Xxmm Family Of Backdoors

C 17 Std Variant Rangarajan Krishnamoorthy On Programming And Other Topics

Sinan This New Clippy Lint Is So Useful I Wish Language Server Ide Implementations Had A Feature For Querying Size Of A Type So That I Could Make Make More Informed Decision

Variant Manager Overview Unreal Engine Documentation

Reference Genome Indexing Times And Index Sizes For Complete Human Download Table
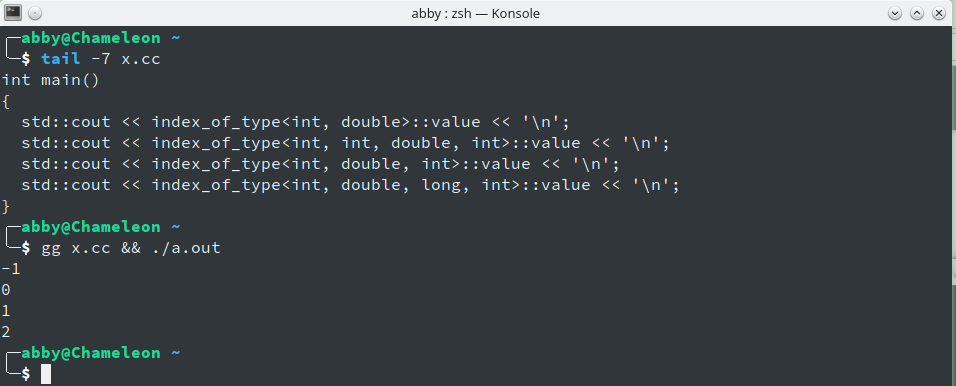
Variant Md
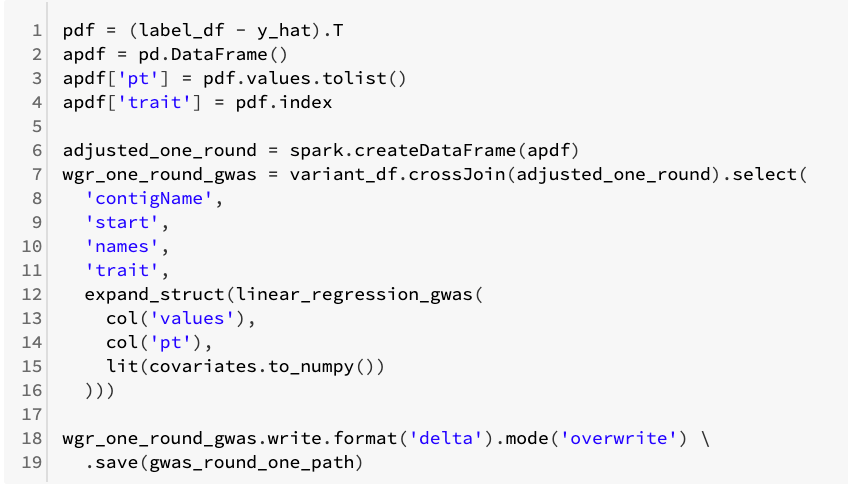
Glowgr Introduction And How To Accelerate Genetic Mixed Models For Genetics With Whole Genome Regression In Glow The Databricks Blog
C Core Guidelines Rules For Strings Modernescpp Com
Repositori Upf Edu Bitstream Handle Gulati An evaluation Pdf Sequence 1 Isallowed Y

C 17 Std Variant Rangarajan Krishnamoorthy On Programming And Other Topics
I Alway Cannot Get The Correct Data By Safearraygetelement

Cr5 Tnzul8ddqm

Std Variant Is Everything Cool About D The D Blog
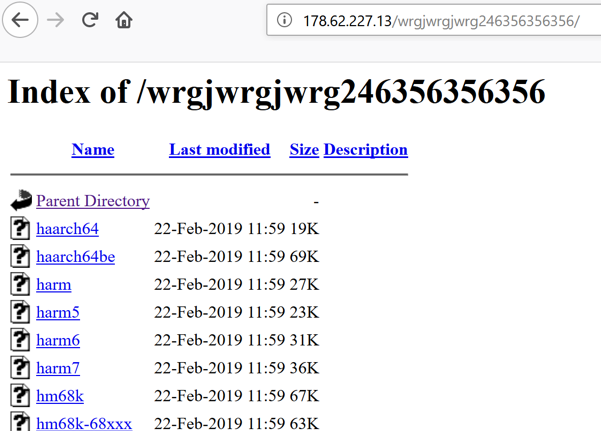
Mirai Botnet Variants Targeting New Processors And Architectures

Variant Condition Propagation With Variant Sources And Sinks Matlab Simulink

Clingen Allele Registry Links Information About Genetic Variants Pawliczek 18 Human Mutation Wiley Online Library

Ncbi C Toolkit Cobjectinfo Class Reference